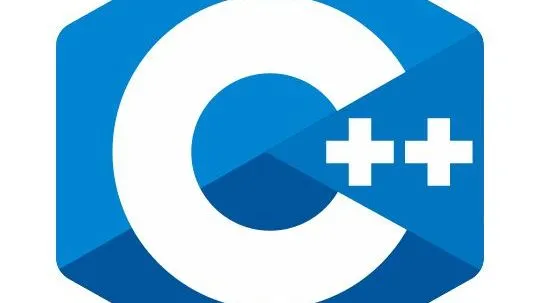
C++ Cheatsheet
Introduction
Hello, in this article I wanted to go over some quick concepts that might help you to better learn and understand the C++ programming language. This will be more focused on game development with C++ than anything as that is what I use the language for. Hopefully you have some fun going over these concepts with me. I tried to keep the examples to game characters and effects. Let's get started.
Expressions
An expression is what we call a piece of code that combines things, returns a value or even calls a function. Not to get too ahead of ourselves with functions but hopefully if you are reading this, you already have some familiarity with another programming language. If not no worries. Just keep reading and will cover concepts like functions later on.
Statements
Statements are pieces of your code where you are declaring things. For instance you can declare two variables. Declaring variables just means giving them the type of data that they will hold as well as the name of the variable.
Variables
You have seen variables before this section but wanted to cover a few more that you might use for your games. Such as the following:
Truthy Values
Truth values are one of two states: true or false. These are also known as truthy values or the more common name which is boolean. Anything with a zero value is false. Some examples:
00 is false,
0.00 is false,
0.000012 is true.
In order for the value to be false it has to contain all zeros.
Relational Operators
Relational operators are used to test whether a given statement is true or false based on the relation of the two items. The operators are:
> - Greater Than
< - Less Than
>= - Greater Than or Equal To
<= - Less Than or Equal To
== - Equal To
!= - Not Equal
Some code examples are below:
I haven't given code examples of them all. Just one of each. If you want to see what happens with say less than or even use the greater than or equal to operator you are more than welcome to. I wanted to give you an option to play around with it yourself to see how they work and try out the different ones on your own.
If Statement
The if statement is used to run specific code blocks or statements if this particular if statement is true. If statements are commonly called conditionals. They are a very handy tool to use in your arsenal especially for game development. Below are some examples:
If/Else Statement
When you need to have two options that each run a piece of code depending on a certain conditon you can use the if/else statement. Below is a code snippet:
Only one of these statements will run. That is why these are called a conditional. If condition one is true then print "You Died!" to the screen, if not then print "You need health badly!"
If/Else if/ Else
When you need to have multiple outcomes based on a condition you can use the else if statement to provide that functionality. The below code example shows this in action:
You can use an if...else if...else to check for certain condtions in your game such as whether or not the player has died and do a certain action according to what state the player is in.
Scope
Scope is a topic that can take a little bit to understand but is kind of simple once everything starts to click. Below we have an example of different scopes with comments denoting where each scope ends and another begins. Let's take a look.
Identifier
An identifier is a name assigned to a program element. Can be any of the following:
Variable
Type
Function
Namespace
Template
Class
All identifiers are case sensitive. This means that an identifier that starts with a lowercase letter isn't the same as one that starts with an uppercase letter and vice versa.